Data structure -Part 2- Stack
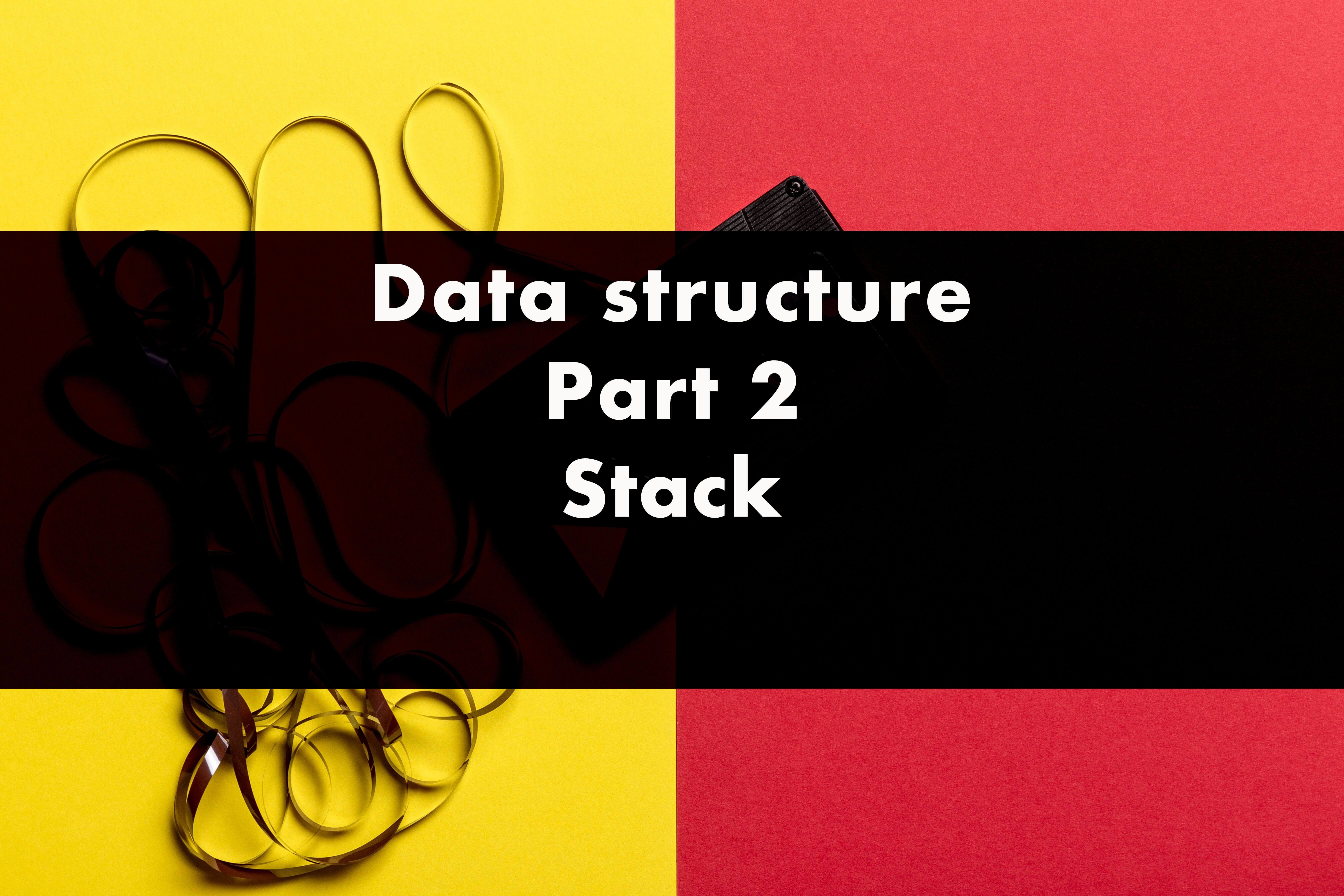
A stack is a list of elements that are accessible only from one end of the list, which is called the top. The stack is known as a last-in, first-out (LIFO) data structure.
Previous parts:
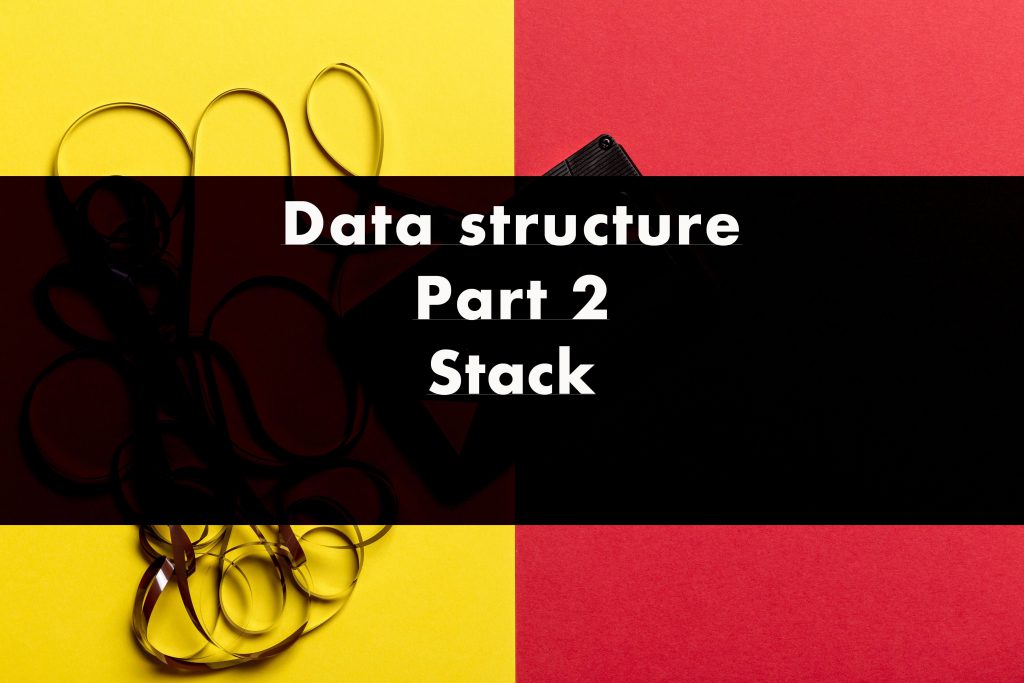
Because of the last-in, first-out nature of the stack, any element that is not currently at the top of the stack cannot be accessed. To get to an element at the bottom of the stack, you have to dispose of all the elements above it first.
The two primary operations of a stack are adding elements to a stack and taking elements off a stack. Elements are added to a stack using the push operation. Elements are taken off a stack using the pop operation.
The peek operation returns the value stored at
the top of a stack without
removing it from the stack.
If you like more structure learning documentation. Please take a look at‘Data-Doc’ online documentation
https://raufr.github.io/datadoc/#/Stacks
Stack Implementation
To build a stack, we first need to decide on the underlying data structure we will use to store the stack elements. We will use an array in our implementation.
Let us begin our stack implementation by defining the constructor function for a Stack class:
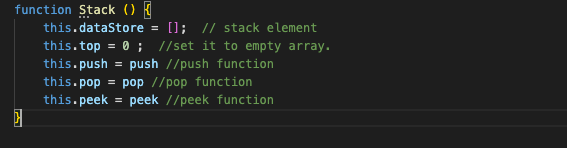
Now we have to implement the push function, also remember, when we have to push new element, we have to store in the top position. The below code can handle this for you.

Pay particular attention to the placement of the increment operator after the call to this.top. Placing the operator there ensures that the current value of top is used to place the new element at the top of the stack before top is incremented.
The pop() function does the reverse of the push() function—it returns the element in the top position of the stack and then decrements the top variable:

The peek() function returns the top element of the stack by accessing the element at the top-1 position of the array:

If you call the peek() function on an empty stack, you get undefined as the result. That’s because there is no value stored at the top position of the stack since it is empty. There will be situations when you need to know how many elements are stored in a stack.
The length() function returns this value by returning the value of top:

Finally, we can clear a stack by simply setting the top variable back to 0:

The below code shows the complete implementation of stack operation.
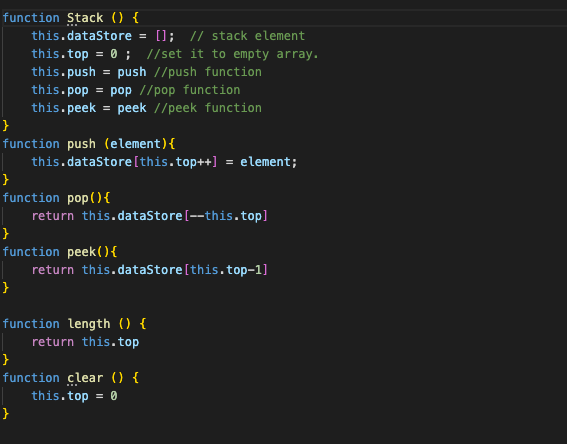
If you may want to test this implementation. The below code can help you.
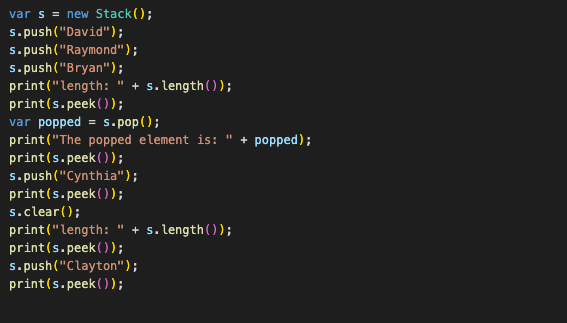
Output:
length: 3
Bryan
The popped element is: Bryan
Raymond
Cynthia
length: 0
undefined
Clayton
Hope you understand the basic of stack operation, There are several problems where stack can be the perfect data structure to solve. Like:
- Multiple Base Conversion
- Palindromes
How to use Stack in Javascript