Data structure-part-1-Array
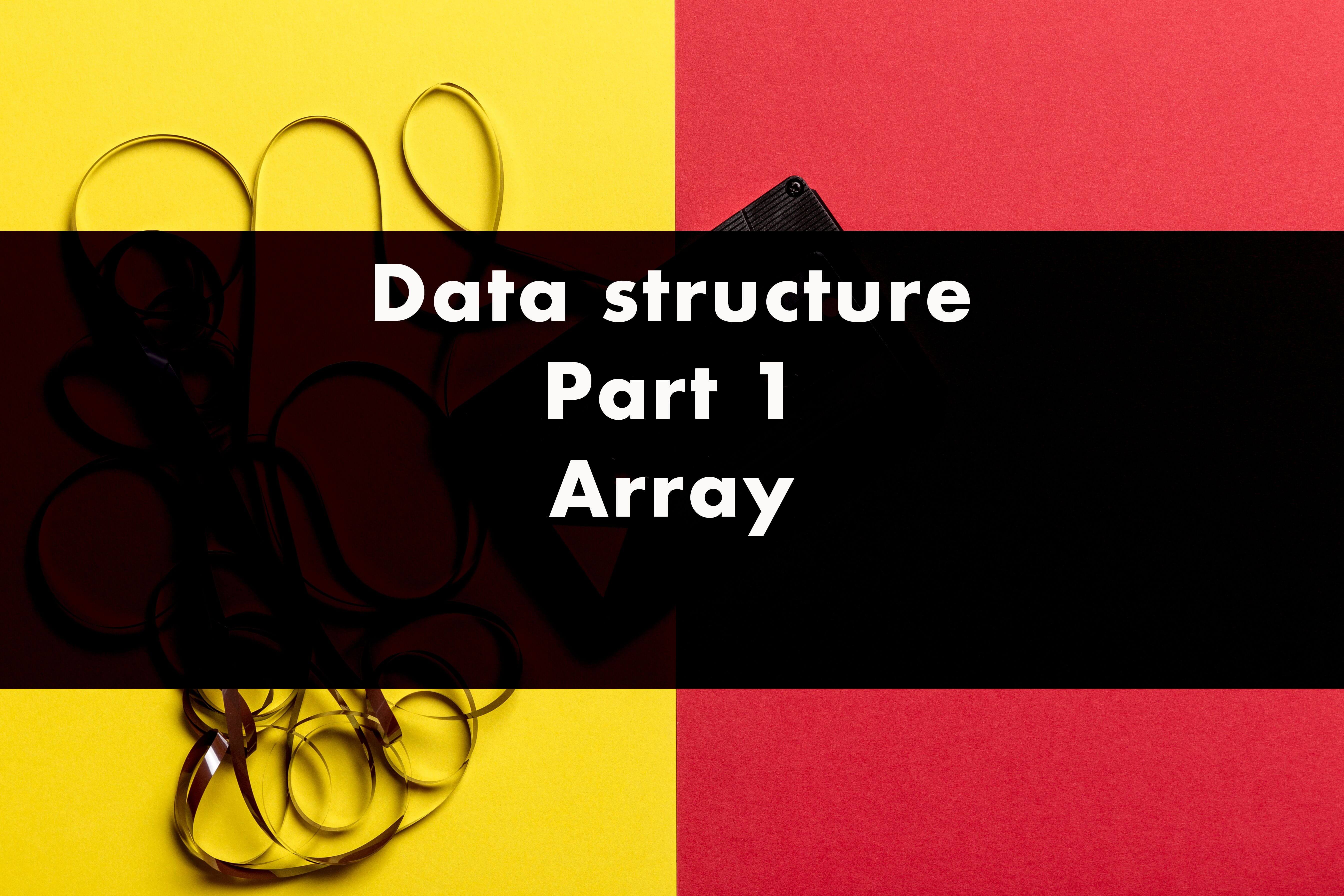
An array is the most common and used data structure in computer programming. This article is part of my data structure series. If you want to know basic about data structure, take a look at the previous part for the introduction.
If you like more structure learning documentation. Please take a look at‘Data-Doc’ online documentation
https://raufr.github.io/datado/Array
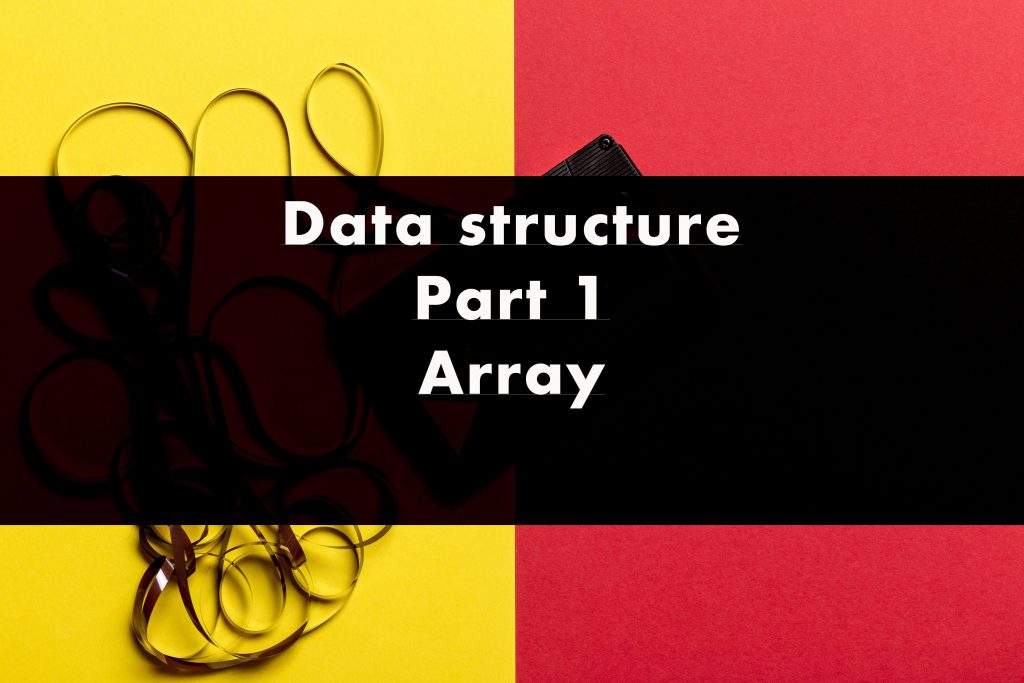
Before starting this tutorial. Please take a glance at the previous part.
This article will focus on array data structure and different ways to implement and use cases. For demonstration purposes, I will use javascript. But use-cases are more-less the same in all languages. Also, It is a good idea for focusing on concept and structure when learning programming basic, rather than focusing on syntax.
Array
Array is a linear collection of elements, where elements can be accessed via indices, which are usually integers used to compute offsets. In javascript array is a specialized type of object, with the indices being property names that cab ve integers used to represent offsets. But internally indices will convert to string to satisfy javascript object requirement
Creating Array
There are some different ways to create an array from scratch.
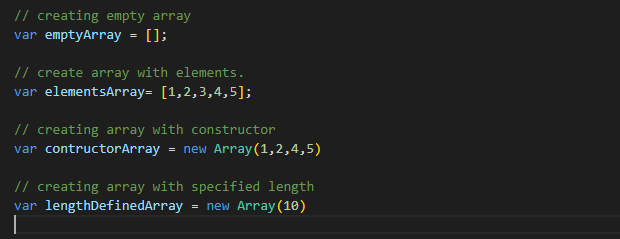
There are other ways to creating arrays from string value or any other object.
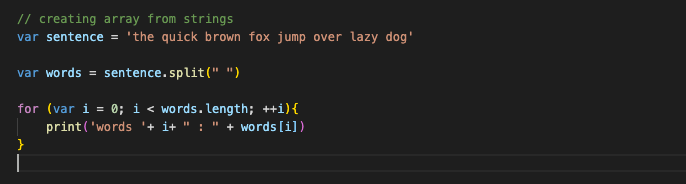
Access & Re-Write Array
You can access and rewrite an array element in several ways. The most computation power saving option is using a loop.
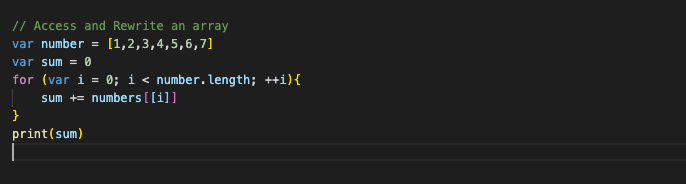
Accessor Functions
Javascript provides some function to access array’ s elements.
indexOf() = Provide index location of an element. If you have multiple occurrences of the same data, indexOf() will return the first position.
lastIndexOf() = Will return last position of string data
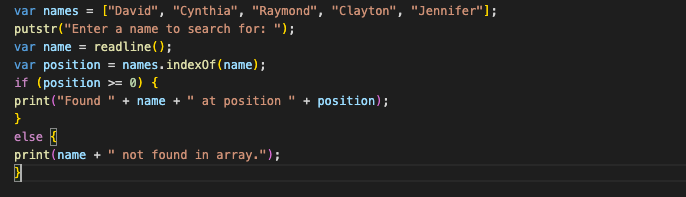
String Representation
There are two functions that return the string representation of an array.
.join()
.toString()
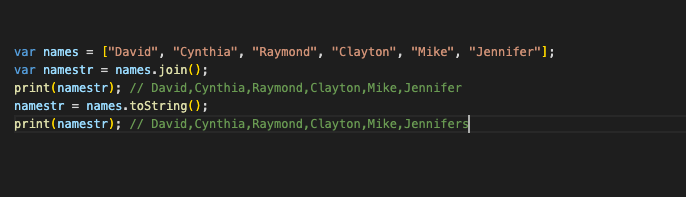
Mutator Functions
Javascript provides some mutator functions that allow you to modify an array.
.push() = Add element to the end of an array

.unshift() = Add element in the begining of an array.
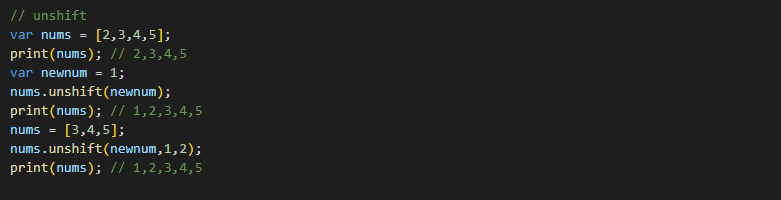
.pop() = Remove element from the end of an array

.shift() =Remove element from begining of an array

.splice() = Adding or removing elements from middle on an array.

.reverese() = Arranging array elements in reverse.

.sort() = Sort array element, this mutator function worked very well with string. With number sort() seems not worked so well due to lexicographucally sorting. Below example is the right way to sort number value with array.sort().
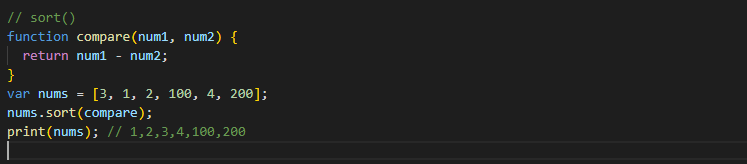
Iterator Functions
There are two types of iterator functions.
- Non-duplication Iterator functions
- Duplication iterator functions
Non-duplication Iterator functions:
forEach() = This function takes a function as an argument and applies the called function to each element of an array.
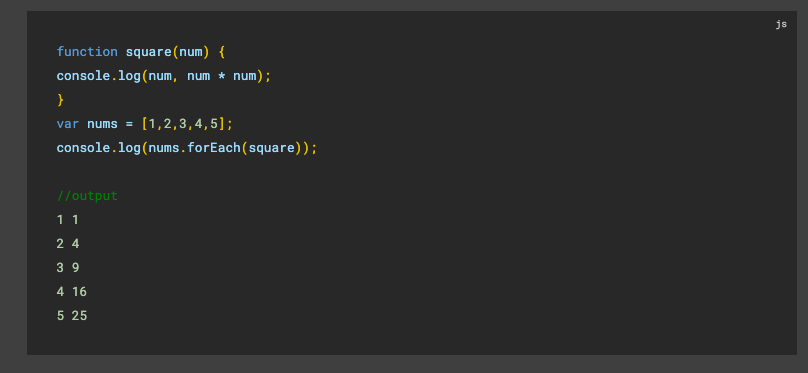
every() = Applies a Boolean function to an array and returns true if the function can return true for every element in the array.
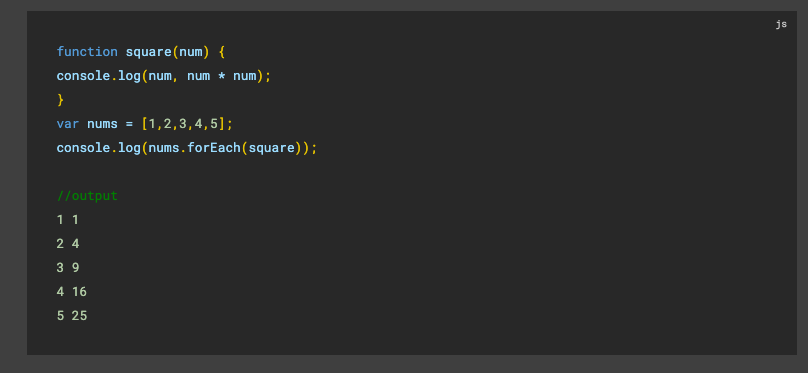
Duplication iterator functions
map() = The map()function works like the forEach() function.
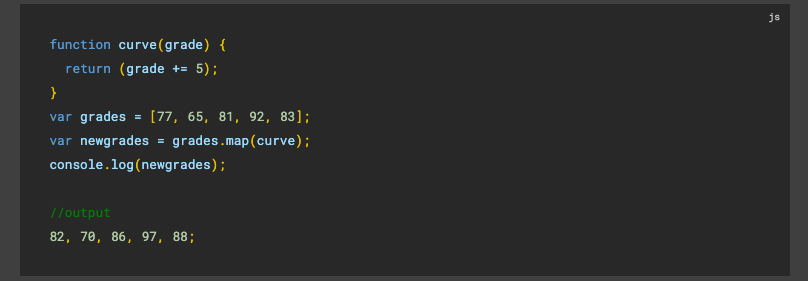
filter() = Work similar as every() function
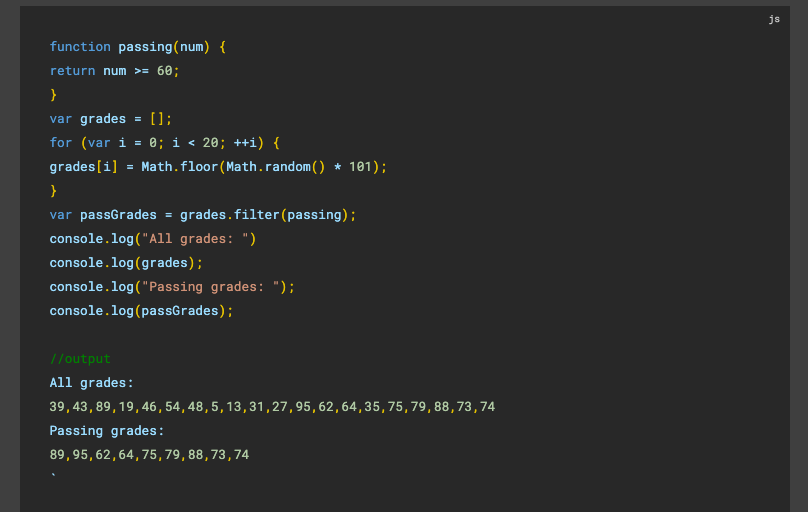
Two Dimentional Array
Creating a 2-dimensional array
By default, javascript does not have a two-dimensional array, but we can do this via creating arrays.
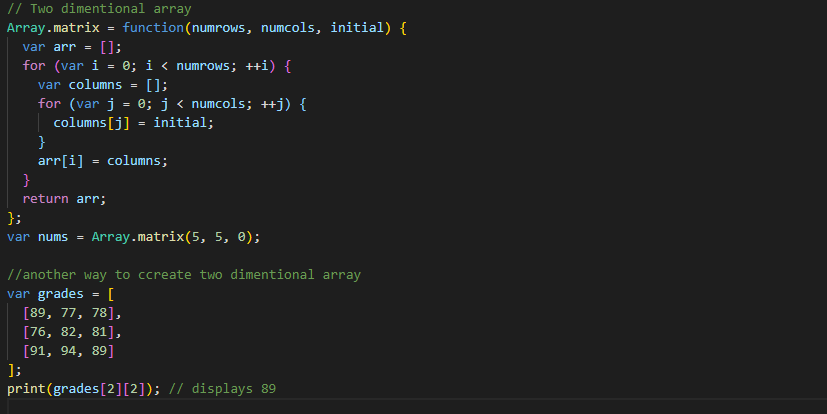
We have just scratch the surface, do not worry
the more we dive in, the more fun it will become.
Happy Coding!