Data structure – Part 3 – Queue
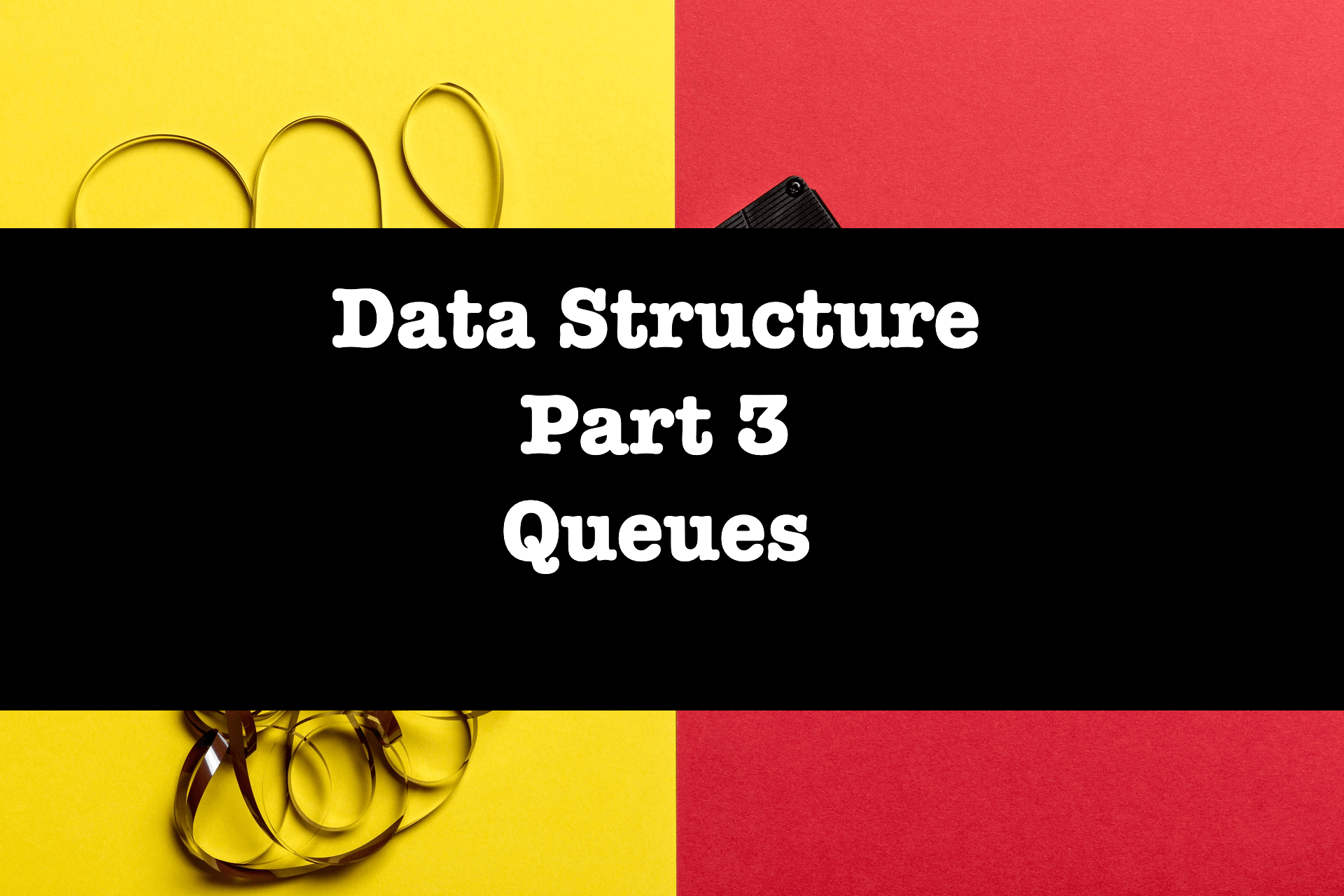
Queues, opposite of Stack data structure and one of the most useful data structures in problem-solving tasks.
A queue is an example of a first-in, first-out (FIFO) data structure. Queues are used to order processes submitted to an operating system or a print spooler, and simulation applications use queues to model scenarios such as customers standing in the line at a bank or a grocery store.
If you like structure documentation! Please go to my official Data-doc where you will find standard development documentation.
https://raufr.github.io/datadoc/#/Queues
Now let’s take a look at queue implementation in Javascript.
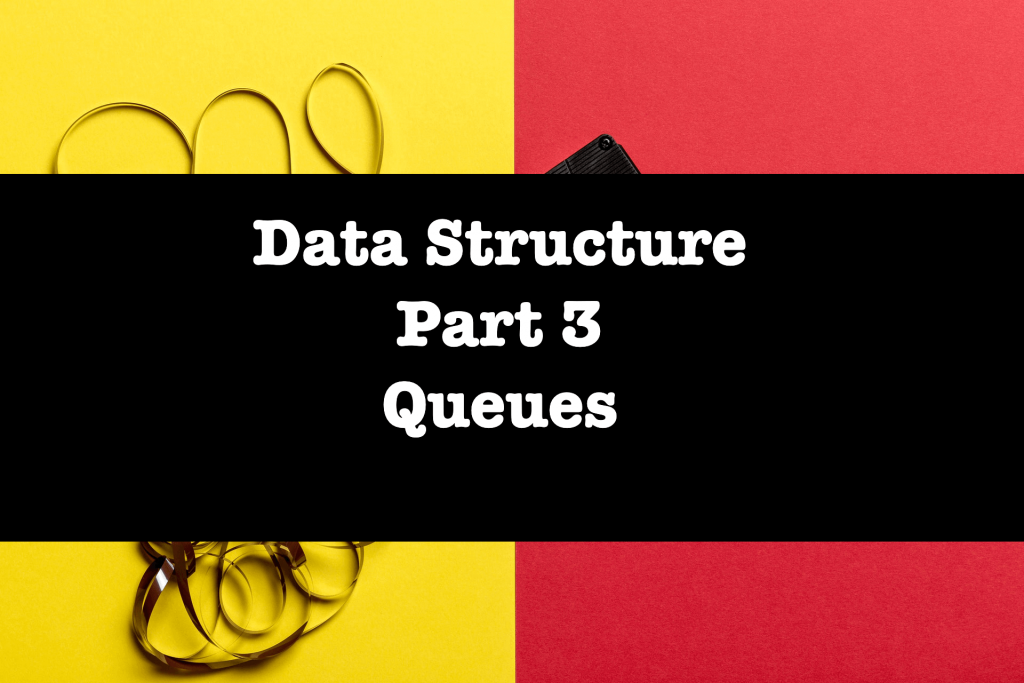
The first thing we have to do, create a bunch of functions for different operations.
Implementing the Queue class using an array is straightforward. Using JavaScript arrays is an advantage many other programming languages don’t have because JavaScript contains a function for easily adding data to the end of an array, push(), and a function for easily removing data from the front of an array, shift().
Before move further, I highly recommend understanding the Array() data structure.
enqueue() and dequeue() functions are depend on push and shift function of array operation. Usually, add elements in a queue in the beginning or end. Very easy to implement. dataStore
is an empty array to holds all the elements.
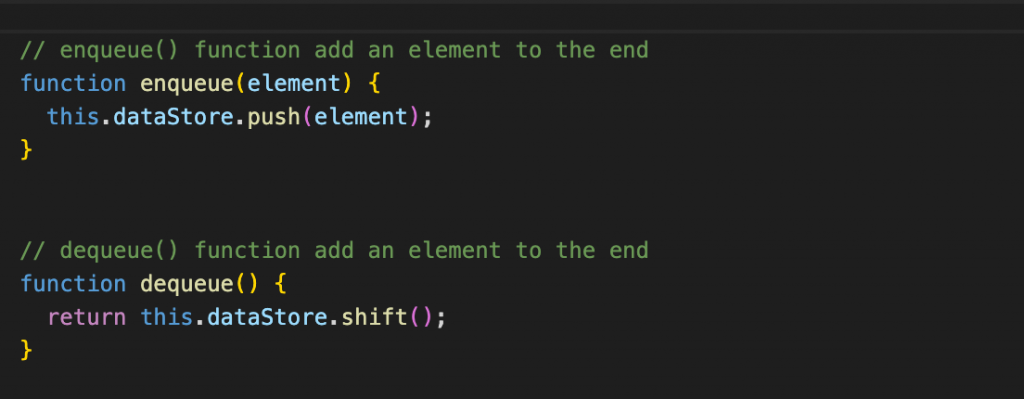
front() and back() function are basically accessible function. Which will return the value of current queue elements.
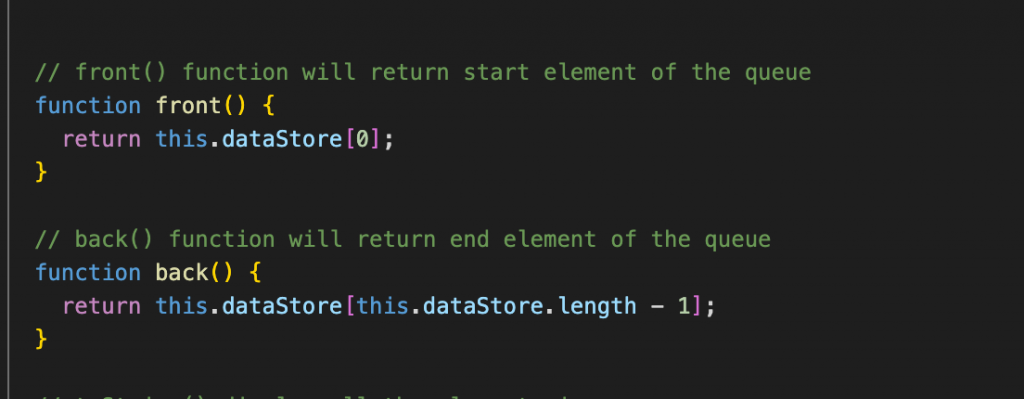
Last two function we need, toString() and empty(). Provide checking functionality in a queue.
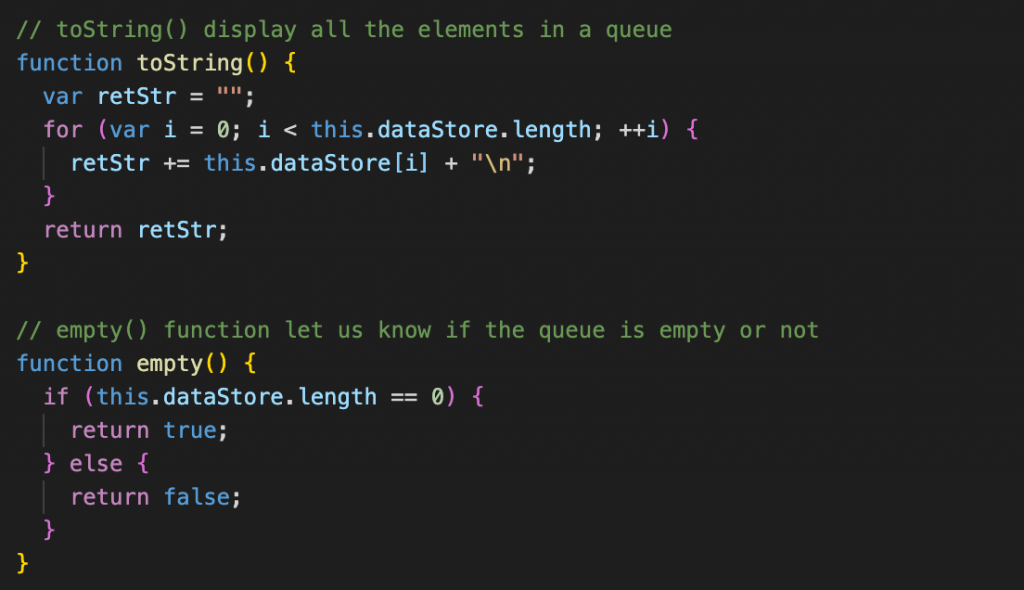
We are done. Right now with the main function, our Queue HOF will look like.
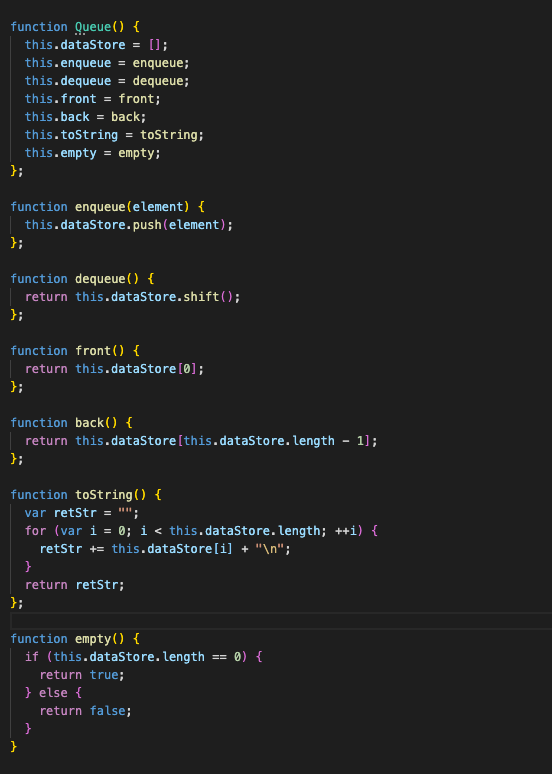
What should we do now 😉
We will test the functionality of our fresh queue.
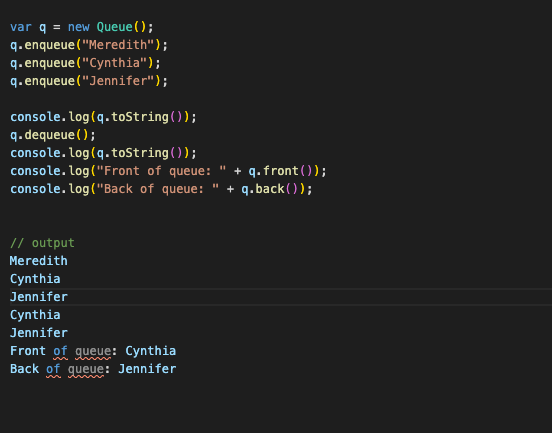
That’s it. In the next article, we will discuss some real-world use case with queue data structure